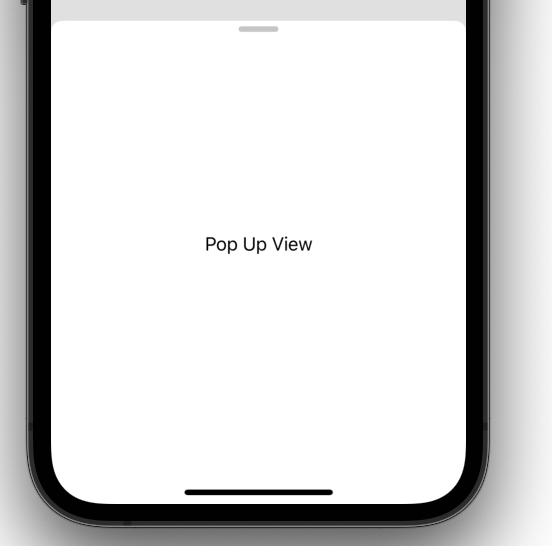
From iOS 16 onwards you can create a popup with inbuilt Apple’s API. i.e. .presentationDetents(
..
Here i have passed values .presentationDetents([.medium, .large])
Means it will show as medium height first then by dragging it it will cover the full height.
struct ContentView: View {
@State var isPresentedPopUp: Bool = false
var body: some View {
Button {
self.isPresentedPopUp.toggle()
} label: {
Text("Present half sheet")
}
.sheet(isPresented: $isPresentedPopUp) {
PopUpView()
.presentationDetents([.medium, .large])
}
}
}
struct PopUpView: View {
var body: some View {
Text("Pop Up View")
}
}
Setting of custom height of Popup –
.presentationDetents([.height(250)]) // use Your value here
Percentage height of view – use .fraction(0.8)
.presentationDetents([.fraction(0.8)]) // 80 Percent of view height
Set Custom Indents
You can create your own detents so that you can set Min height & Max Height of the Popup. See the FromDetent & ToDetent
struct ContentView: View {
@State var isPresentedPopUp: Bool = false
var body: some View {
Button {
self.isPresentedPopUp.toggle()
} label: {
Text("Present half sheet")
}
.sheet(isPresented: $isPresentedPopUp) {
PopUpView()
.presentationDetents([.custom(FromDetent.self), .custom(ToDetent.self)])
}
}
}
struct PopUpView: View {
var body: some View {
Text("Pop Up View")
}
}
struct FromDetent: CustomPresentationDetent {
static func height(in context: Context) -> CGFloat? {
return 100
}
}
struct ToDetent: CustomPresentationDetent {
static func height(in context: Context) -> CGFloat? {
return 200
}
}
Any suggestion ? You can write Comments 👇🏽