[Question] : Given a 2D matrix, your task is to rotate the matrix 90 degrees clockwise.
Input: – [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Output – [[7, 4, 1], [8, 5, 2], [9, 6, 3]]
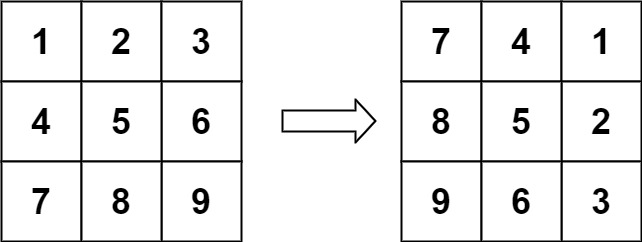
func rotateMatrix(_ matrix: inout [[Int]]) {
var rows = matrix.count
var columns = rows
//transpose of Matrix
for row in 0..<rows {
for column in row..<columns {
let temp = matrix[row][column]
matrix[row][column] = matrix[column][row]
matrix[column][row] = temp
}
}
for row in 0..<rows {
matrix[row].reverse()
}
}
var matrixToRotate = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
rotateMatrix(&matrixToRotate);
print("Rotated matrix output-->", matrixToRotate)// [[7, 4, 1], [8, 5, 2], [9, 6, 3]]