[Question]: Given a positive integer number N. The task is to generate all the binary strings of N bits. These binary strings should be in ascending order.
Example: –
Input: 2
Output:
0 0
0 1
1 0
1 1
func generateAllBinaryStrings(_ n: Int, _ arr: inout [Int], _ i: Int) {
if i == n {
print("Exit: Binary arr-------------------------------->", arr)
return
}
print("** First Recursion Here>", i, "_ array", arr)
// First assign "0" at ith position and try for all other permutations for remaining positions
arr[i] = 0
generateAllBinaryStrings(n, &arr, i + 1)
// And then assign "1" at ith position and try for all other permutations for remaining positions
arr[i] = 1
print("-- Second Recursion Here>", i, "_ array", arr)
generateAllBinaryStrings(n, &arr, i + 1)
}
var lengthOfBinary = 2
var binArry:[Int] = Array.init(repeating: 0, count: lengthOfBinary)
generateAllBinaryStrings(lengthOfBinary, &binArry, 0)
Output -->
** First Recursion Here> 0 _ array [0, 0]
** First Recursion Here> 1 _ array [0, 0]
Exit: Binary arr--------------------------------> [0, 0]
-- Second Recursion Here> 1 _ array [0, 1]
Exit: Binary arr--------------------------------> [0, 1]
-- Second Recursion Here> 0 _ array [1, 1]
** First Recursion Here> 1 _ array [1, 1]
Exit: Binary arr--------------------------------> [1, 0]
-- Second Recursion Here> 1 _ array [1, 1]
Exit: Binary arr--------------------------------> [1, 1]
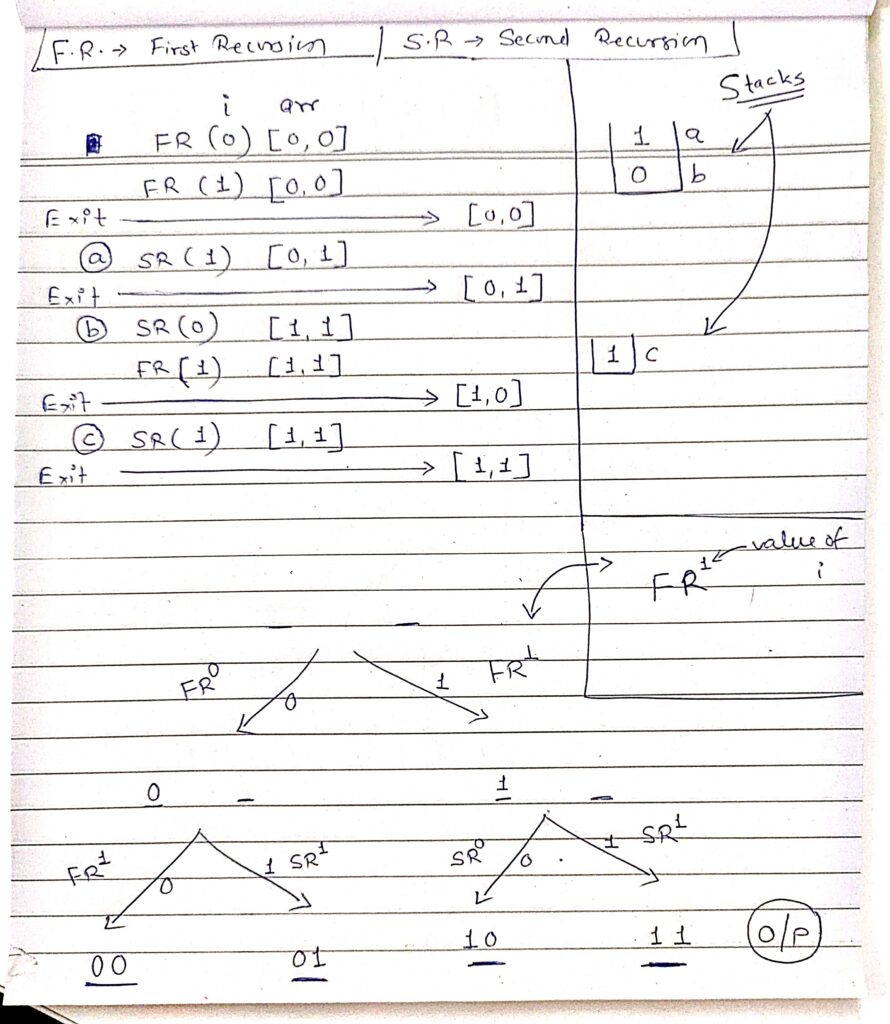