To populate a list of items in the iPhone you can use UITableView
which provides vertical scrolling over the items. In this article we will UITableView
in iOS with storyboard
and add some items to it.
Let’s Code
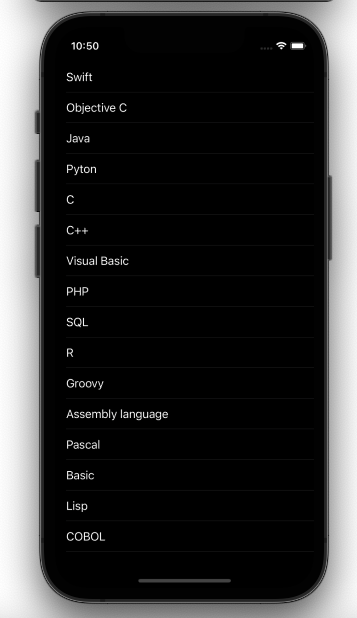
Step 1: Create New Xcode Project & jump towards Main.StoryBoard
Step 2: Click on + icon(Object Library) & Drag UITableView
to StoryBoard
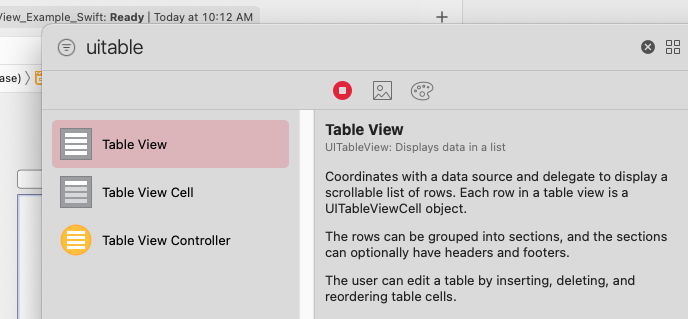
Step 3: Add leading, trailing top bottom
constraints to the tableView
, deselect the Constraint to margin Option.
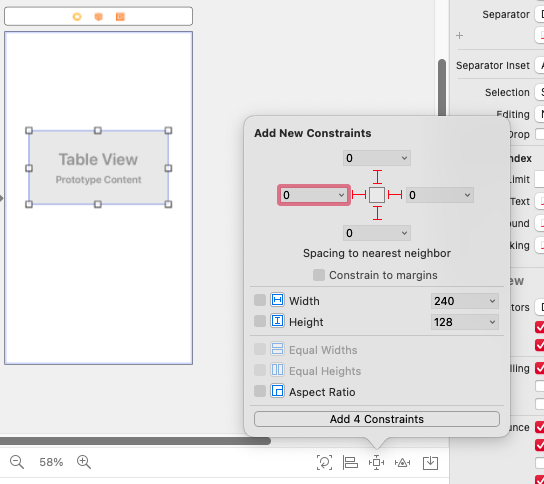
Step 4: Create a IBOutlet
of UITableView
. By clicking right click on UITableView
& drag the line to ViewController.swift f
ile
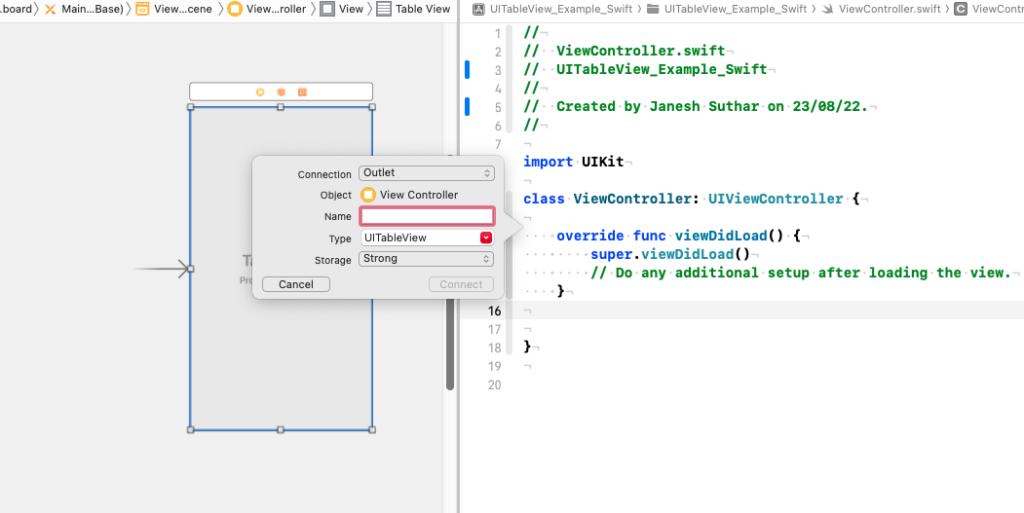
Step 5: To link Data/Array to the UItableView we have Make dataSource conformance to self Means The current ViewController class will adopt the methods of UITableViewDataSouce & we can provide data objects to the UITableView
– numberOfRowsInSection
used for how many rows to present in UITableView
–cellForRowAt
used for designing the cell items
Step 6: To listen click events of UITableView we have Make delegate conformance to self
The Complete Code is here –
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var customTableView: UITableView!
var programmingLanguagesArray = ["Swift", "Objective C", "Java", "Pyton", "C", "C++", "Visual Basic", "PHP", "SQL", "R", "Groovy", "Assembly language", "Pascal", "Basic", "Lisp", "COBOL"]
override func viewDidLoad() {
super.viewDidLoad()
setUpUItableView()
}
// Setting UItableview
func setUpUItableView() {
customTableView.dataSource = self
customTableView.delegate = self
customTableView.estimatedRowHeight = 80// To make resizable cells
customTableView.rowHeight = UITableView.automaticDimension
//To register Fresh cell to the TableView
customTableView.register(UITableViewCell.self, forCellReuseIdentifier: "cell")
}
}
// MARK: UITableViewDataSource
extension ViewController: UITableViewDataSource {
// Return a list of item in array
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return programmingLanguagesArray.count
}
// return a cell for specific row
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "cell") else {
return UITableViewCell()
}
var content = cell.defaultContentConfiguration()
content.text = programmingLanguagesArray[indexPath.row]
cell.contentConfiguration = content
return cell
}
}
// MARK: UITableViewDelegate
extension ViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
print("Clicked on row -->", indexPath.row)
}
}
Here is Apple reference
A complete Source code is Here : )