iOS 16 onwards
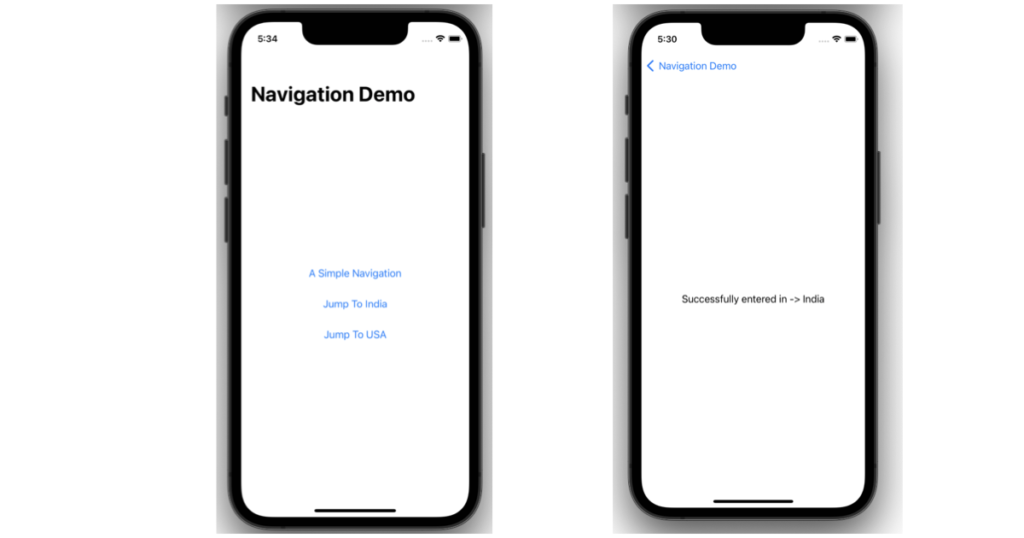
User NavigationStack
for top Header(NavView) & use NavigationLink
for jump to next view –
struct NavigationViewExample: View {
var body: some View {
NavigationStack {
VStack {
NavigationLink {
Text("Click Here")
} label: {
Text("Detail View")
}
}
.navigationTitle("Navigation iOS 16")
}
}
}
Additionally you can use .navigationBarTitleDisplayMode(.inline)
for lesser height NavigationBar
Use Of .navigationDestination
To jump with specific value use navigationDestination
let me explain you in simple way by giving Genuine example –
Create a Hashable
model in Swift – where hash(into
is used for Hashable conformance & static func == (lhs
is for Equatable protocol & countryName
is property
class CountryModel: Hashable, Equatable {
static func == (lhs: CountryModel, rhs: CountryModel) -> Bool {
lhs.countryName == rhs.countryName
}
func hash(into hasher: inout Hasher) {
hasher.combine(countryName)
}
var countryName: String = ""
init(title: String) {
self.countryName = title
}
}
Now we can use navigationDestination
with CountryModel which is Hashable –
struct NavigationViewExample: View {
let countryList: [CountryModel]
var body: some View {
NavigationStack {
VStack(spacing: 30) {
NavigationLink {
DetailView()
} label: {
Text("A Simple Navigation")
}
NavigationLink(value: CountryModel.init(title: "India")) {
Text("Jump To India")
}
NavigationLink(value: CountryModel.init(title: "USA")) {
Text("Jump To USA")
}
}
.navigationDestination(for: CountryModel.self, destination: { currentStuff in
Text("Successfully entered in -> " + currentStuff.countryName)
})
.navigationTitle("Navigation Demo")
}
}
}
struct DetailView :View {
var body: some View {
Text("This is Detail View")
}
}
Any suggestion ? comments are welcomed 👇🏽👇🏽👇🏽