[Question]: Given a string s
, find the length of the longest substring without repeating characters.
Example 1:
Input: s = "abcabxy" Output: 5 Explanation: The answer is "abcxy", with the length of 5
func lengthOfLongestSubstring(_ s: String) -> Int {
var longest = 0, startIndex = 0
var charMap: [Character: Int] = [:]
for (index, char) in s.enumerated() {
if let foundIndex = charMap[char] {
startIndex = max(foundIndex + 1, startIndex)
}
longest = max(longest, index - startIndex + 1)
charMap[char] = index
}
return longest
}
let longestSubStrInput = "abcabxy"
let outputOfLongest = lengthOfLongestSubstring(longestSubStrInput)
//print(" output of longest substring without repeating chars", outputOfLongest) // OP->5
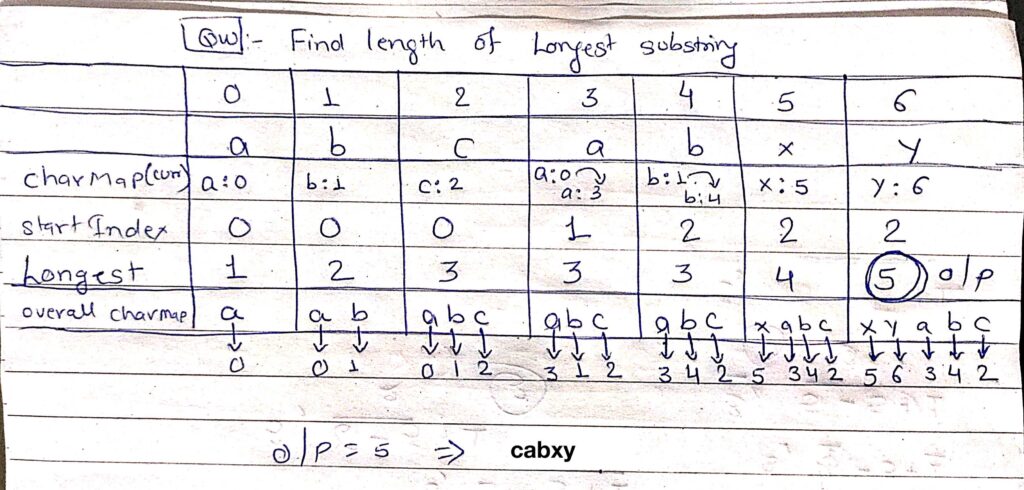