[Question] Given the head
of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return the reordered list.
The first node is considered odd, and the second node is even, and so on.
You must solve the problem in O(1)
extra space complexity and O(n)
time complexity.
Example 1:
Input: head = [1,2,3,4,5] Output: [1,3,5,2,4]
func oddEvenList(_ head: ListNode?) -> ListNode? {
var oddHead = head
var evenHead = head?.next
var evenOrignalHead = head?.next
while evenHead != nil && evenHead?.next != nil {
oddHead?.next = evenHead?.next // Step A
oddHead = oddHead?.next // Step B
evenHead?.next = oddHead?.next// Step C
evenHead = evenHead?.next// Step D
}
oddHead?.next = evenOrignalHead // Step E
return head
}
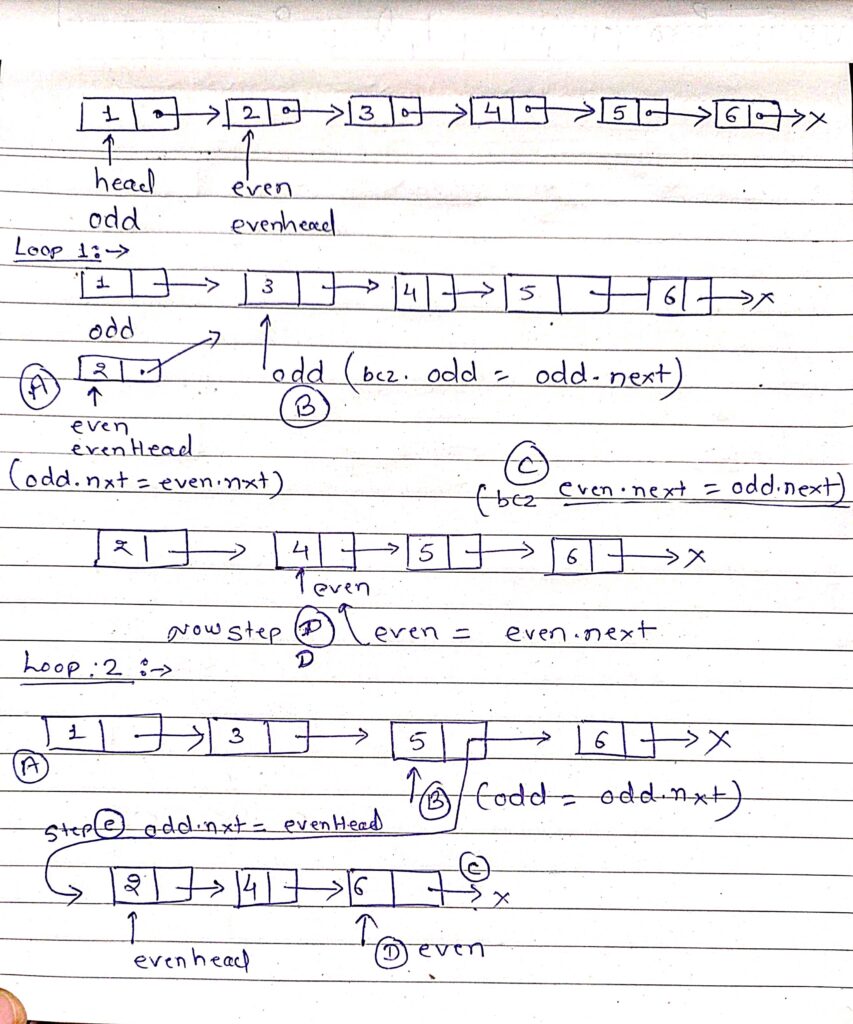