[Question] Reverse Singly Link List using Iterative & Recursion approach.
// Iterative Approach
func reverseList1(_ head: ListNode?) -> ListNode? {
var prev = head, currentHead = head?.next
prev?.next = nil
while currentHead != nil {// At end of linked list it will be nil.
let next = currentHead!.next
currentHead!.next = prev
prev = currentHead
currentHead = next
}
return prev
}
// Recursive Approach
func reverseList2(_ head: ListNode?) -> ListNode? {
if head == nil || head?.next == nil {
return head
}
let newHead = reverseList2(head?.next)
var nextNode = head?.next
nextNode?.next = head
head?.next = nil
return newHead
}
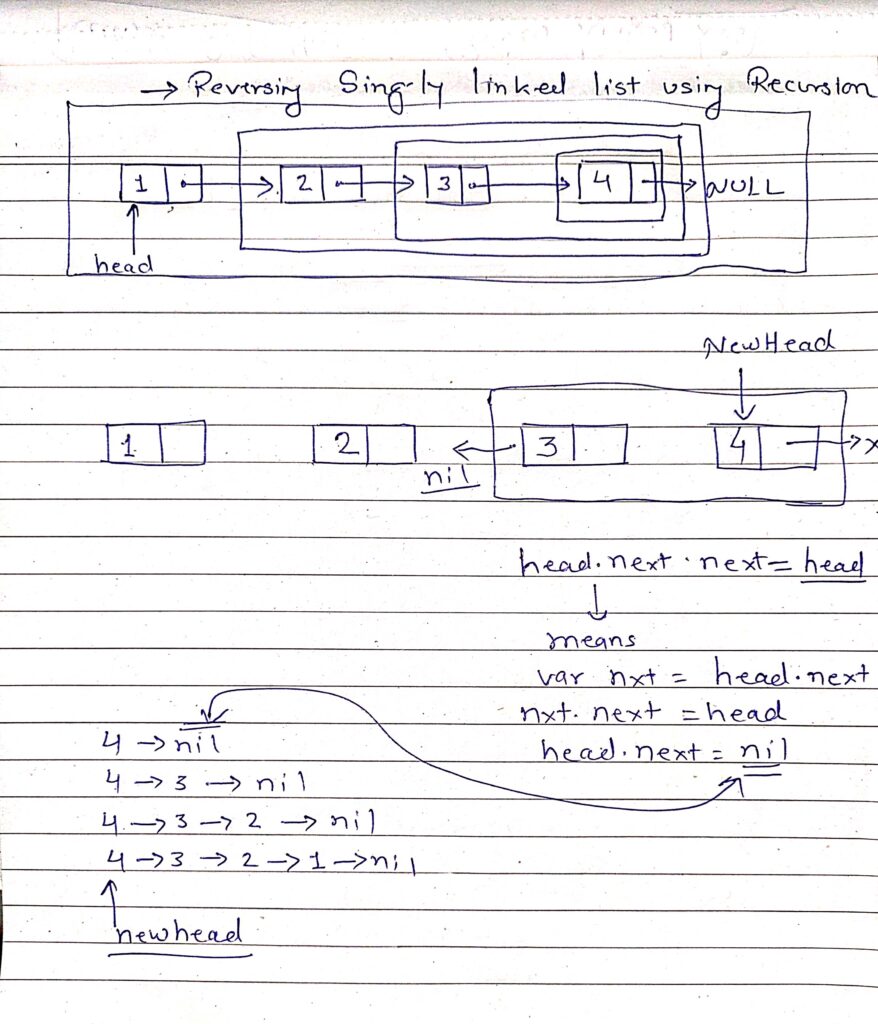