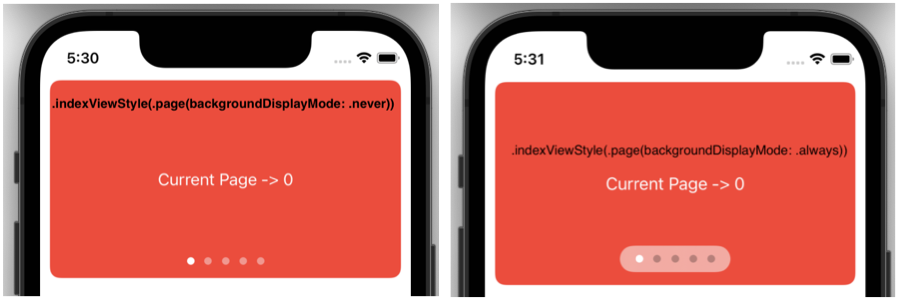
Just Like a UIPager
in Swift, we will use TabView
in SwiftUI to create a page effect with dot indicator on it.
TabView
switches between multiple childviews So we can swipe around and it will jump to the next item.- Here we will be using the
ForEach
which is used to add multiple items over it. ZStack
is used for just a background effect..tabViewStyle(.page)
is used to create dot indicator pager effect- Optional –
indexViewStyle(.page(backgroundDisplayMode: .never))
when we use never it will create no background effect as shown in reference image 1.If we use.always
it will show a background around the pager. You can try.interactive
and play around. 🙂
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
PagerInSwiftUI()
Spacer()
}
}
}
struct PagerInSwiftUI: View {
var body: some View {
TabView {
ForEach(0..<5) { i in
ZStack {
Color.red
Text("Current Page -> \(i)").foregroundColor(.white)
}.clipShape(RoundedRectangle(cornerRadius: 10.0, style: .continuous))
.frame(height:200)
}
.padding(.all, 10)
}
.frame(width: UIScreen.main.bounds.width, height: 200)
.tabViewStyle(.page)
.indexViewStyle(.page(backgroundDisplayMode: .never))// To set indexStyle(Background of pager)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
A more example to embed into complex UI is here – https://janeshswift.com/ios/swiftui/how-to-create-complex-ui-with-swiftui/